生活札记
Java学习笔记 - 基础入门(一)
Java 是一个通用术语,用于表示 Java 软件及其组件,包括“Java 运行时环境 (JRE)”、“Java 虚拟机 (JVM)”以及“插件”。 Java具有大部分编程语言所共有的一些特征,被特意设计用于互联网的分布式环境。Java具有类似于C++语言的形式和感觉,但它要比C++语言更易于使用,而且在编程时彻底采用了一种以对象为导向的方式。
教程:https://www.bilibili.com/video/BV1rB4y1G7WE
Java下载:https://www.oracle.com/java/technologies/downloads/#jdk20-windows
Java IDE IntelliJ:https://www.jetbrains.com.cn/idea/download/#section=windows
环境配置:
添加环境变量:path 下添加java的bin目录 C:\Program Files\Java\jdk1.8.0_361\bin
添加常量:JAVA_HOME:C:\Program Files\Java\jdk1.8.0_361
查看版本:java -version
java version "1.8.0_361"
Java(TM) SE Runtime Environment (build 1.8.0_361-b09)
Java HotSpot(TM) 64-Bit Server VM (build 25.361-b09, mixed mode)
一、基础
1)、二进制转十进制:0 0 0 0 1 1 1 1 = 0 + 0 + 0 + 0 + 1(8) + 1(4) + 1(2) + 1(1) = 15
2)、十进制转二级制:15转二级制
15/2 = 7 余数 1
7/2 = 3 余数 1
3/2 = 1 余数 1
1/2 = 0 余数 1
结果:0 0 0 0 1 1 1 1
3)、数据类型:
4)、数组:
int[] scores = {1,2,3,4,5};
int[] scores = new int[3];
scores[0] = 1;
scores[1] = 2;
scores[2] = 3;
System.out.println("scores = " + Arrays.toString(scores));
//定义
int[] scores = new int[5];
//系统输入
Scanner scanner = new Scanner(System.in);
for (int i = 0; i < 5; i++) {
scores[i] = scanner.nextInt();
}
//排序
Arrays.sort(scores);
System.out.println("scores = " + Arrays.toString(scores));
5)、类、字符串、随机数、递归、正则表达式:
package copylian.java;
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Student {
//属性
String stdName;
int age;
String[] course;
/**
* 获取学生信息
* @author copylian@aikehou.com
* @date 2023年6月4日23:20:14
* @return String[]
*/
private String getStdName(){
return "学生:" + stdName + " , 年龄:" + age + " 岁, 学习了课程:" + Arrays.toString(course);
}
/**
* 获取学生其他信息
* @author copylian@aikehou.com
* @date 2023年6月4日23:20:14
* @return String[]
*/
public String[] getCourse(){
String info = getStdName();
String[] arr = new String[]{info, "其他信息"};
return arr;
}
/**
* 递归
* @param num
* @return
*/
public static int digui(int num){
if (num == 1){
return num;
} else {
return num * digui(num - 1);
}
}
/**
* main
* @param args
*/
public static void main(String[] args){
//实例化
Student student = new Student();
student.stdName = "只袄早";
student.age = 20;
student.course = new String[]{"GoLnag","PHP","Python","Java"};
String info = student.getStdName();System.out.println("info = " + info);
String[] other = student.getCourse();
System.out.println("Arrays.toString(other) = " + Arrays.toString(other));
//字符、字符串:
char a = 'A'; // 字符只能是一个
String b = "ABCD";
System.out.println("a = " + a);
System.out.println("b = " + b);
System.out.println("b.equals(a) = " + b.equals(a));
//字符串操作
b.concat();
b.trim();
b.split();
b.substring();
b.indexOf();
b.lastIndexOf();
//StringBuffer() - 多线程、StringBuilder() - 单线程
Scanner input = new Scanner(System.in);
String str = input.next();
StringBuffer strbuf = new StringBuffer(str);
System.out.println("strbuf = " + strbuf);
strbuf.append("Java"); // 附加
System.out.println("strbuf = " + strbuf);
strbuf.insert(0,"aaa"); // 插入
System.out.println("strbuf = " + strbuf);
//随机数
double math = Math.random();
System.out.println("math = " + math); // 很短时间内可能出现重复
Random r = new Random();
Double r1 = r.nextDouble();
System.out.println("r1 = " + r1);
int r2 = r.nextInt(100);
System.out.println("r2 = " + r2);
//递归
int dg = digui(5);
System.out.println("dg = " + dg);
//正则表达式
String regex = "^1[345789]\\d{9}";
Pattern p = Pattern.compile(regex);
Matcher m = p.matcher("15859279220");
System.out.println("m.matches() = " + m.matches());
System.out.println("m.find() = " + m.find());
while(m.find()){
System.out.println("m.group() = " + m.group()); // m.group() 获取匹配的字序列
}
}
}
6)、Java OOP封装:封装、继承(extends)、多态、抽象类(abstract)、接口类(interface),接口类继承(implements),基本上跟PHP的类似,这里就不详细赘述了
import copylian.java.oop.Animal;
import java.util.Arrays;
public class Testoop {
public static void main(String[] args){
String type = "狗东西";
int age = 10;
String[] hobbys = new String[]{"抓老鼠","出去玩"};
Animal animal = new Animal(type, age, hobbys);
System.out.println("animal = " + animal.getType() + animal.getAge() + Arrays.toString(animal.getHobbys()));
}
}
//Animal.java类
package copylian.java.oop;
import copylian.java.Student;
public class Animal extends Student {
//类型
private String type;
//age
public int age;
//爱好
protected String[] hobbys;
//颜色
public static String color;
/**
* 构造函数:可以同时存在多个同名的构造函数,但是参数得不一样
* @param type
* @param age
* @param hobbys
*/
public Animal(String type, int age, String[] hobbys) {
//super父类
super();
this.type = type;
this.age = age;
this.hobbys = hobbys;
}
/**
* 同名构造方法
* @param type
* @param age
*/
public Animal(String type, int age) {
this.type = type;
this.age = age;
}
/**
* 静态方法:不能访问非静态属性
*/
public static void print(){
System.out.println("color = " + color);
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String[] getHobbys() {
return hobbys;
}
public void setHobbys(String[] hobbys) {
this.hobbys = hobbys;
}
}
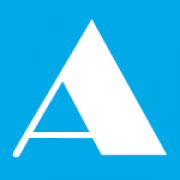
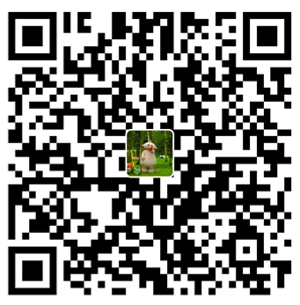
文明上网理性发言!